Start and Exit the SDK
The SDK has to be started before using and also has to be exited after using it. The methods start() and exit() are asynchronous method calls to do this.
sdk.start();
After the SDK is successfully started it will fire an onStarted() event of the SDKListener, or an onExited() event if it is exited successfully. In case of an error it will fire an onStartError() or an onExitError() event.
class MySDKListener implements SDKListener {
public void onStarted() {
System.out.println("sdk was successfully started...");
}
public void onExited() {
System.out.println("sdk was successfully exited...");
}
public void onPlug() {}
public void onUnplug() {}
}
public class MySDKErrorListener implements SDKErrorListener {
public void onStartError( ErrorEventObject error) {
System.out.println("error occured when starting sdk...");
}
public void onExitError( ErrorEventObject error) {
System.out.println("error occured when exiting sdk...");
}
public void onGeneralError(ErrorEventObject error) {}
public void onDeviceError(ErrorEventObject error) {}
}
To shut down the sdk use the method exit().
sdk.exit();
Another possibility to start and exit the SDK are the synchronous methods startAndWait() and exitAndWait(). These methods will block the executing thread until the SDK was successfully started or exited. In case of an error they will throw a SDKStartException or a SDKExitException.
try {
sdk.startAndWait();
} catch (SDKStartException e) {
// handle the start exception here...
}
// use some SDK functions here...
try {
sdk.exitAndWait();
} catch (SDKExitException e) {
// handle the exit exception here...
}
The SDK States
It is possible to request the current state of the sdk with the method getState(). The value SDKState.STARTED will indicate that the sdk is ready to use. The following illustration shows the complete state machine of the sdk.
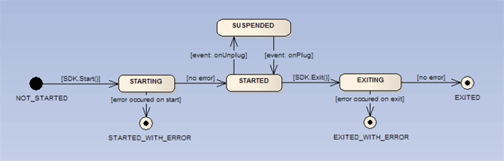
Illustration 1 - State machine of the SDK states